Dynamic Menubar Item
After login in, an application shows a dynamic menubar item with information about the authenticated user, typically in the top-right corner where the Sign in button lives for unauthenticated users.
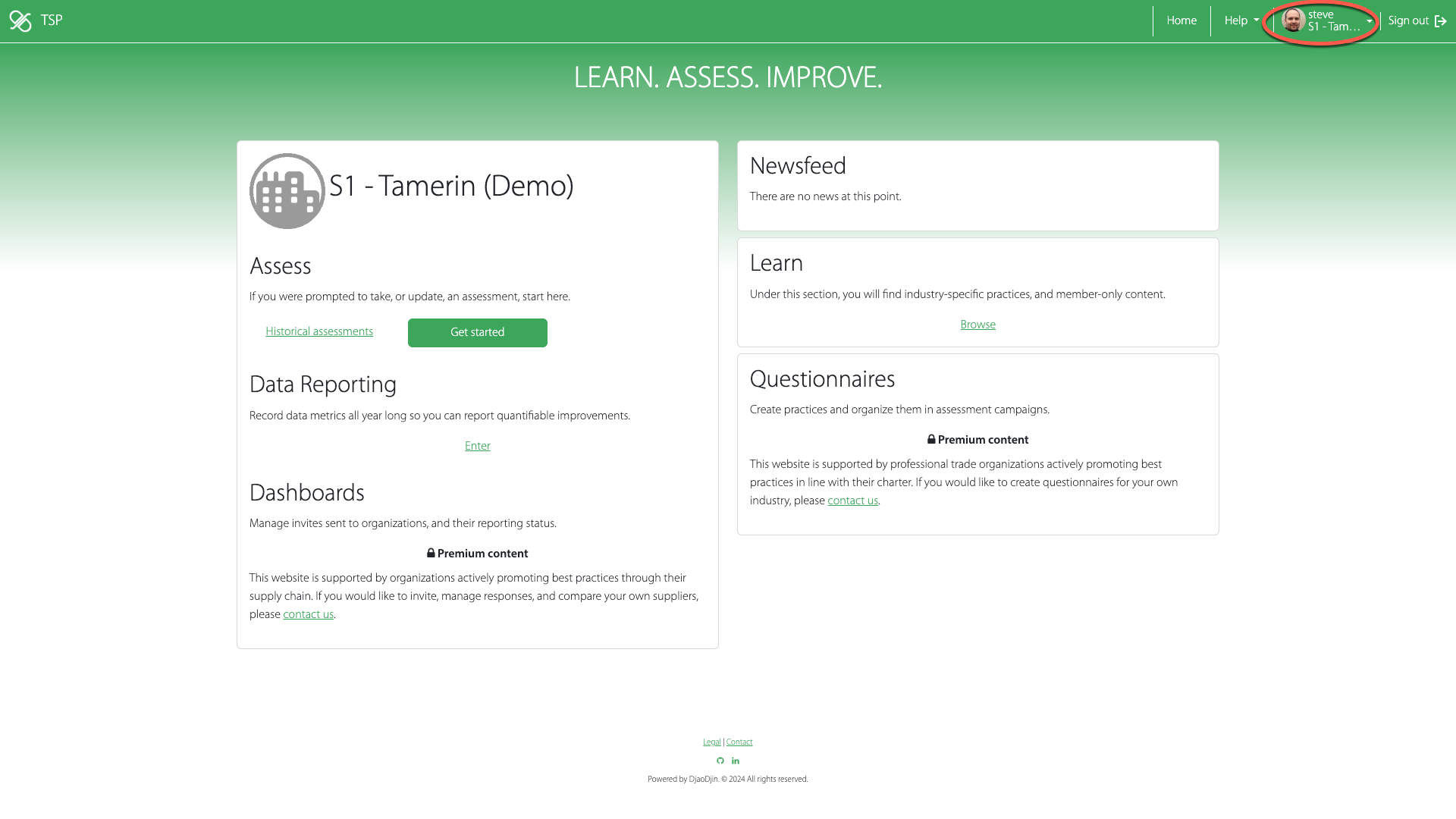
Relying on theme templates
When you rely on theme templates, DjaoApp will automatically
replace the content within the HTML node matching attribute
data-dj-menubar-user-item
with a dropdown menu to access
a user and connected profiles application home and settings pages.
By adding the following code into your template, the authenticated user drop-down menu will appear in-place.
<head>
...
+ <link rel="stylesheet" media="screen" href="//mysite.djaoapp.com/assets/cache/djaodjin-menubar.css" />
</head>
<body>
...
+<div data-dj-menubar-user-item>
+ <a id="login" href="/login/">Sign In</a>
+</div>
...
+<script type="text/javascript" src="//mysite.djaoapp.com/assets/cache/djaodjin-menubar.js"></script>
</body>
</html>
You can customize the dropdown menu by editing the _menubar.html template.
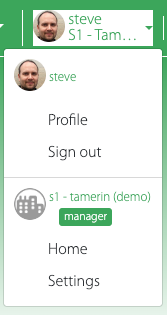
Relying on API calls
If you are running a Single Page App, or hosting your pages outside DjaoDjin, you will rely on API calls instead of the DjaoApp proxy to inject the dynamic manubar item into the HTML response.
The simplest way to add a dynamic menubar item through pages hosted
outside DjaoDjin is to add a data-dj-menubar-user-item
node
element load in your HTML page, as well as load djaodjin-menubar.css and
djaodjin-menubar.js as done in the example above.
The second way to add a dynamic menubar item through the API is to call /api/users/{user} and /api/users/{user}/accessibles, then construct an HTML element from the JSON responses of these API endpoints.
If the pages you want to inject the dynamic menubar item into are served from
a different domain than your DjaoDjin site (i.e. mysite.djaoapp.com),
you will need to take into consideration Cross-origin resource sharing (CORS) rules.
That means, in particular, that if you call
/api/auth?cookie=1
from your own domain to authenticate users, you will need to pass
credentials: 'include' to fetch
otherwise
the cookies won't be set (see Set-Cooking).
async function authUser(event) {
// Prevents the form to be submitted to the server
// through the `action` attribute.
event.preventDefault();
// Fetch the user credentials from the form input fields
const username = event.target.querySelector('[name="username"]').value
const password = event.target.querySelector('[name="password"]').value
// Call the authentication API
const data = {'username': username, 'password': password};
const resp = await fetch("mysite.djaoapp.com/api/auth?cookie=1", {
method: "POST",
credentials: 'include',
headers: {'Content-Type': 'application/json'},
body: JSON.stringify(data)
})
if( resp.status == 201 ) {
// Extract the JWT and decode the user account information.
const respData = await resp.json();
const authToken = respData.token;
sessionStorage.setItem('authToken', authToken);
// Move on to the authenticated part of the application...
injectUserMenubarItem();
}
return 0;
}
UI Considerations
If you are going to modify the default _menubar.html template, or build the HTML for a dynamic menubar item from sratch, you will need to remember the following corner cases:
- Very long user and profile names
- Ridiculously high number of connected profiles
- Missing user and profile pictures
- Need help?
- Contact us
- Curious how it is built?
- Visit us on GitHub